PayPal
- Initiate Authorization
- Collect Payment Option Details via SDK
- PayPal Checkout
- Payment Confirmation
- Complete Authorization
1. Initiate Authorization
The authorization of a payment is initialized by calling the API method 1.38 Init Authorize.
In the example below, we authorize 3.99 EUR for a PayPal payment as part of the purchase of two premium widgets from Widgets GmbH. The shopper's full name is provided alongside their address.
Initiate Authorization Request
Path:
PUT {baseURL}/payment/initAuthorize
Header:
Content-Type: application/json
Accept-Language: en-US
X-Auth-Token: eyJhbGciOiJSUzI1NiI{abbreviated}RW5kVG9rZW4=
{
"partnerReference": "DEV-SVR001-DE_CUSTID-DYTTXCPK9B_CARTID-6TYF3XT4FC_V4MMDX34JG",
"programAccno": "1679541175",
"accno": "MERCHANT-DE4321",
"accnoType": "00",
"paymentOptionCode": "PAYPAL",
"presentationAmount": 3.99,
"presentationCurrCode": "EUR",
"presentationUsage": "Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95.",
"useDifferentBillingAddress":true,
"customerFullName": "Jacob Smith",
"addr1":"Anystreet",
"houseNumber":"321",
"city":"Anycity",
"countryCode":"DE",
"postCode":"12345",
"emailAddress": "user@example.com",
"localDate": "2018-10-28",
"localTime": "124735",
"custom1": "WVWZZZ3BZWE689725",
"criteria": [
{
"name": "returnURL",
"value": "https://example.com/Payment/CompleteAuthorization"
},
{
"name": "cancelURL",
"value": "https://example.com/Payment/CancelAuthorization"
}
]
}
In contrast to other payment options, the usage of the parameter "emailAddress" is mandatory for PayPal payments. The "returnUrl" and "cancelUrl" (in the "criteria"-object) specify, where the customer is redirected after a successful or aborted payment, respectively.
Please note only URLs starting with "https://" are allowed as values of the "returnURL" and "cancelURL" sub-parameters (in the "criteria" object)
It is strongly advised that real customer information be accurately provided - i.e., "useDifferentBillingAddress" being set to true, making the below mandatory:
customerFullName
addr1
houseNumber
(if not included in "addr1")city
postCode
countryCode
It is advised that "emailAddress" also be provided, with accurate customer data.
Please discuss with you business contacts when providing accurate data may not be possible in production, as it may result in transactions being rejected.
If the value of "countryCode" is "US" (United States of America) or "CA" (Canada) the "state" parameter is required. The value of "state" must be a valid State Code. (ex. "countryCode": "US", "state": "NY")
The maximum character length of the presentation usage (see variable presentationUsage
in the example above) varies between payment options. It may be that with certain payment options the specified presentation usage may be less than 127 and consequently be truncated. It would thus be strongly recommended that the most pertinent information be placed at the beginning of the presentation usage. To be compatible with most payment options we suggest that the presentation usage already be truncated at the 22nd character.
Initiate Authorization Response
Status Code:
201 (Created)
Header:
Content-Type: application/json
Accept-Language: en-US
{
"programAccno": "1679541175",
"accno": "MERCHANT-DE4321",
"uniqueReference": "zdPGHjinWUmwEe82uIA-eA",
"loadAccountReference": "NvPoE85cZE6FguOfrC7Fmw",
"authorizationToken": "PAY-75U79999CW5902937LPK2COQ",
"paymentOptionCode": "PAYPAL",
"presentationAmount": 3.99,
"presentationCurrCode": "EUR",
"presentationUsage": "Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95."
"custom1": "WVWZZZ3BZWE689725",
"statusCode": "RECEIVED",
"statusReason": "created",
"paymentProviderResponse": {
"id": "PAY-75U79999CW5902937LPK2COQ",
"intent": "authorize",
"state": "created",
"payer": {
"payment_method": "paypal"
},
"transactions": [
{
"amount": {
"total": "3.99",
"currency": "EUR"
},
"description": "Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95.",
"invoice_number": "zdPGHjinWUmwEe82uIA-eA",
"payment_options": {
"allowed_payment_method": "UNRESTRICTED",
"recurring_flag": false,
"skip_fmf": false
},
"related_resources": []
}
],
"create_time": "2018-10-28T11:44:57Z",
"links": [
{
"href": "https://api.sandbox.paypal.com/v1/payments/payment/PAY-75U79999CW5902937LPK2COQ",
"rel": "self",
"method": "GET"
},
{
"href": "https://www.sandbox.paypal.com/cgi-bin/webscr?cmd=_express-checkout&token=EC-5UF50497VT869573A",
"rel": "approval_url",
"method": "REDIRECT"
},
{
"href": "https://api.sandbox.paypal.com/v1/payments/payment/PAY-75U79999CW5902937LPK2COQ/execute",
"rel": "execute",
"method": "POST"
}
]
},
"partnerReference": "DEV-SVR001-DE_CUSTID-DYTTXCPK9B_CARTID-6TYF3XT4FC_4Q7TQTC2HX",
"localDate": "2018-10-28",
"localTime": "124454",
"sysDate": "2018-10-28",
"sysTime": "114458",
"responseCode": "0000",
"responseDescription": "Successful execution",
"additionalInformation": {
"requestId": "aff2728481a181dc36daedc14055b516"
}
}
The response includes the desired authorization token under the return parameter "authorizationToken" which is used to collect payment option details via the SDK and alongside the "uniqueReference" is required to complete the authorization of the initiated transaction. The transaction reference under the return parameter "uniqueReference" is furthermore required for the following API calls. Beyond this use, it should be persisted if possible, as it enables the identification of the transaction should the need arise at a later stage.
2. Collect Payment Option Details via SDK
The SDK renders a customizable PayPal button and transmits all necessary payment details to start the PayPal Checkout flow. We offer SDKs for the integration into websites as well as mobile applications (Android and iOS).
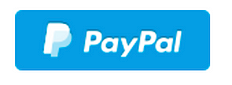
The example below shows only the relevant part of the KC Web SDK implementation for Guest Payments with PayPal. A complete and detailed description of the KC Web SDK can be found here.
The authorization token returned by the API method 1.38 Init Authorize which initiated the transaction is used to associate the payment option details collected via the KC Web SDK with the transaction.
Collect Payment Option Details via KC Web SDK
// Initialize Payment Form renderer options.
cw.initialize({
enablePayPal: true // Required for PayPal provider.
});
// Configure and render the Payment Form
cw.PaymentForm(container,
{
authorizationToken: "PAY-75U79999CW5902937LPK2COQ",
paymentOptionCodes: ["PAYPAL"],
paymentProviderMode: "test",
paypalButtonStyle: { // optional
size: "medium", // default "small"
color: "blue", // default "gold"
shape: "rect", // default "pill"
label: "paypal", // default "paypal"
tagline: "false" // default "false"
}
});
A detailed guide how to customize the PayPal button is provided on the PayPal Developer Portal.
3. PayPal Checkout
PayPal displays a login page, but keeps the customer local to your website or mobile app throughout the payment process.
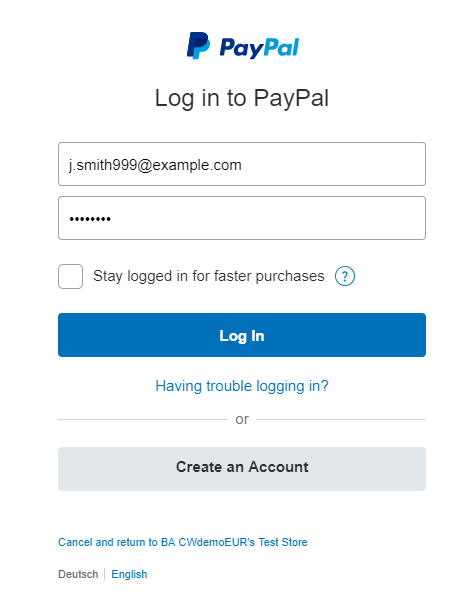
The customer can either directly enter their login credentials or create a new PayPal account. In the latter case, they must specify a new payment option (e.g., credit card or bank account).
4. Payment Confirmation
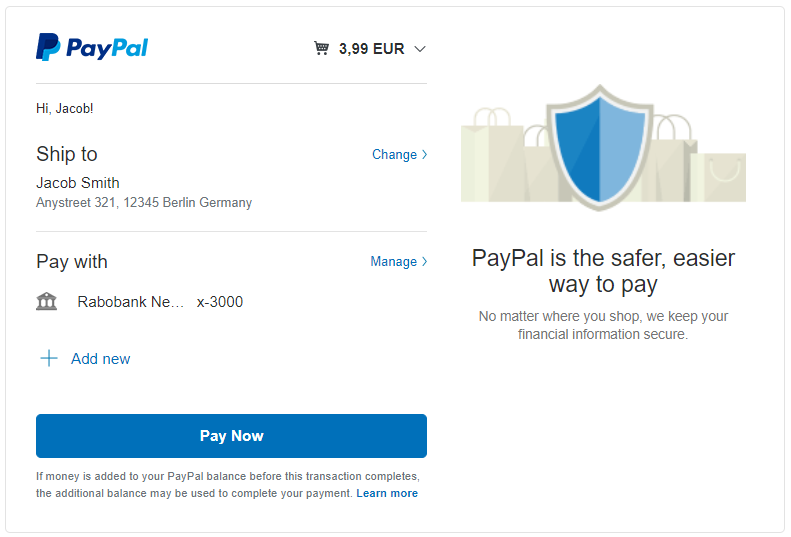
Following this, the Welcome back page shows the billing address, the chosen payment option and the amount to be authorized. Clicking the cart symbol displays the payment details (see parameter "presentationUsage" in the Initiate Authorization Request).
After confirming the payment, the shopper is redirected to the "returnUrl".
5. Complete Authorization
Since the SDK sends the payment details directly to PayPal, where the payment is subsequently processed, you have no knowledge about the current status of the payment and if it got authorized. Therefore, call the API method 1.39 Complete Authorize from your server-side method behind the "returnURL", which you specified in the SDK.
Complete Authorization Request
Path:
POST {baseURL}/payment/{uniqueReference}/completeAuthorize
POST {baseURL}/payment/zdPGHjinWUmwEe82uIA-eA/completeAuthorize
Header:
Content-Type: application/json
Accept-Language: en-US
X-Auth-Token: eyJhbGciOiJSUzI1NiI{abbreviated}RW5kVG9rZW4=
{
"partnerReference": "DEV-SVR001-DE_CUSTID-DYTTXCPK9B_CARTID-6TYF3XT4FC_V4MMDX34JG",
"authorizationToken": "PAY-75U79999CW5902937LPK2COQ",
"useDifferentBillingAddress":true,
"customerFullName": "Jacob Smith",
"addr1":"Anystreet",
"houseNumber":"321",
"city":"Anycity",
"countryCode":"DE",
"postCode":"12345",
"localDate": "2018-10-28",
"localTime": "124735",
"criteria": [
{
"name": "PayerID",
"value": "FJFBNSGR8YTBC"
}
]
}
If the value of "countryCode" is "US" (United States of America) or "CA" (Canada) the "state" parameter is required. The value of "state" must be a valid State Code. (ex. "countryCode": "US", "state": "NY",)
The transaction authorization is identified by the "uniqueReference" and "authorizationToken" returned initially by the API method 1.38 Init Authorize. Instead of saving these variables on your server-side, pass them via the query string parameters in the "returnUrl".
In addition, PayPal uses the "PayerID" to identify a transaction, which is attached as a query string parameter to the "returnUrl" and must be specified in the "criteria"-object.
Complete Authorization Response
Status Code:
200 (OK)
Header:
Content-Type: application/json
Accept-Language: en-US
{
"initiatorAccno": "1679541175",
"accno": "1679797975",
"uniqueReference": "zdPGHjinWUmwEe82uIA-eA",
"initiationCountryCode": "DE",
"initiationCountryCode3": "DEU",
"processedAmount": 3.99,
"processedCurrCode": "EUR",
"statusCode": "AUTHORIZED",
"statusReason": "authorized",
"paymentProviderResponse": {
"id": "PAY-75U79999CW5902937LPK2COQ",
"intent": "authorize",
"state": "approved",
"cart": "5UF50497VT869573A",
"payer": {
"payment_method": "paypal",
"status": "UNVERIFIED",
"payer_info": {
"email": "user@example.com",
"first_name": "Jacob",
"last_name": "Smith",
"payer_id": "FJFBNSGR8YTBC",
"shipping_address": {
"recipient_name": "Jacob Smith",
"line1": "Anystreet 321",
"city": "Anycity",
"state": "",
"postal_code": "12345",
"country_code": "DE"
},
"country_code": "DE"
}
},
"transactions": [
{
"amount": {
"total": "3.99",
"currency": "EUR",
"details": {}
},
"payee": {
"merchant_id": "73RGZ6595XXDJ",
"email": "facilitator@example.com"
},
"description": "Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95."
"invoice_number": "zdPGHjinWUmwEe82uIA-eA",
"item_list": {
"shipping_address": {
"recipient_name": "Jacob Smith",
"line1": "Anystreet 321",
"city": "Anycity",
"state": "",
"postal_code": "12345",
"country_code": "DE"
}
},
"related_resources": [
{
"authorization": {
"id": "5FM71539D33965344",
"state": "authorized",
"amount": {
"total": "3.99",
"currency": "EUR",
"details": {}
},
"payment_mode": "INSTANT_TRANSFER",
"reason_code": "AUTHORIZATION",
"protection_eligibility": "ELIGIBLE",
"protection_eligibility_type": "ITEM_NOT_RECEIVED_ELIGIBLE,UNAUTHORIZED_PAYMENT_ELIGIBLE",
"parent_payment": "PAY-75U79999CW5902937LPK2COQ",
"valid_until": "2018-11-26T12:47:14Z",
"create_time": "2018-10-28T11:47:14Z",
"update_time": "2018-10-28T11:47:14Z",
"links": [
{
"href": "https://api.sandbox.paypal.com/v1/payments/authorization/5FM71539D33965344",
"rel": "self",
"method": "GET"
},
{
"href": "https://api.sandbox.paypal.com/v1/payments/authorization/5FM71539D33965344/capture",
"rel": "capture",
"method": "POST"
},
{
"href": "https://api.sandbox.paypal.com/v1/payments/authorization/5FM71539D33965344/void",
"rel": "void",
"method": "POST"
},
{
"href": "https://api.sandbox.paypal.com/v1/payments/authorization/5FM71539D33965344/reauthorize",
"rel": "reauthorize",
"method": "POST"
},
{
"href": "https://api.sandbox.paypal.com/v1/payments/payment/PAY-75U79999CW5902937LPK2COQ",
"rel": "parent_payment",
"method": "GET"
}
]
}
}
]
}
],
"create_time": "2018-10-28T11:47:15Z",
"links": [
{
"href": "https://api.sandbox.paypal.com/v1/payments/payment/PAY-75U79999CW5902937LPK2COQ",
"rel": "self",
"method": "GET"
}
]
},
"partnerReference": "DEV-SVR001-DE_CUSTID-DYTTXCPK9B_CARTID-6TYF3XT4FC_K9FPCYWPJF",
"localDate": "2018-10-28",
"localTime": "124712",
"sysDate": "2018-10-28",
"sysTime": "114715",
"responseCode": "0000",
"responseDescription": "Successful execution",
"additionalInformation": {
"requestId": "aff2728481a181dc36daedc14055b516"
}
}
Note, that the 1.39 Complete Authorize response includes the internal representation of the Account Number indicated by the parameter Account Number Type. Additionally, the response includes the transaction status under the status code parameter, which, at this stage, should be set to AUTHORIZED
, indicating that the payment has been successfully authorized.
Please also see transaction status handling for non-successful response codes.