Google Pay
- Initiate Authorization
- Collect Payment Option Details via KC UPCF Web SDK
- Google Pay™ Checkout and Payment Confirmation
- Complete Authorization
1. Initiate Authorization
The authorization of a payment is initialized by calling the API method 1.38 Init Authorize.
In the example below, we authorize 100 EUR for a Google Pay™ payment as part of the purchase of two premium widgets from Widgets GmbH.
Initiate Authorization Request
Path:
PUT {baseURL}/payment/initAuthorize
Header:
Content-Type: application/json
Accept-Language: en-US
X-Auth-Token: eyJjb25uZW***AwMjZ2PTQifQ==
User-Agent: ***
{
"partnerReference": "eb9640b0-f731-4abf-8752-37e8280c9ee9",
"programAccno": "7228894817",
"accno": "7228905548",
"accnoType": "01",
"presentationAmount": 100,
"presentationCurrCode": "EUR",
"presentationUsage": "V Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95.",
"useDifferentBillingAddress": false,
"paymentOptionCode": "GGLPAY",
"criteria": [
{
"name": "googleButtonType",
"value": "plain"
},
{
"name": "googleButtonStyle",
"value": "white"
}
],
"localDate": "2025-01-28",
"localTime": "125849"
}
The parameter useDifferentBillingAddress
determines whether customer account data is loaded from the technical account. If set to false, the system will automatically obtain billing address data from the technical account. If set to true, the data must be explicitly provided in the request at the root level. Below is an example demonstrating how these parameters should be structured:
"useDifferentBillingAddress": true,
"customerFullName": "Jacob Smith",
"emailAddress": "user@example.com",
"addr1": "Gotzkowskystraße 2332",
"city": "Delbrück",
"countryCode": "DE",
"postCode": "33129",
"phone": "12345678910"
Customizable Google Pay™ Button
To customize the Google Pay™ button within the request, include the googleButtonType
and googleButtonStyle
parameters in criteria
(see example above). The "type" parameter defines the text displayed on the button, such as a standard payment label or a subscription-related prompt, while "style" controls the button's visual theme to match different background and branding requirements. These parameters determine how the Google Pay™ button is generated on the page, ensuring flexibility in its presentation. By specifying these attributes, merchants can align the button's appearance with their checkout flow while maintaining compliance with Google's UI guidelines.
For more details, please refer to the Button Customization documentation.
Multiple Domains Support
To support multiple merchant domains for Google Pay™, merchants need to register them with Google, as only the domains added to Google Pay's whitelist will be valid for use. The registered domain automatically extends to its sub-directories as listed below, allowing Google Pay™ transactions on localized pages without requiring separate configurations.
your-shop.com
your-shop.com/de
your-shop.com/fr
Merchants must align with their Product Solution Specialist to ensure all relevant domains are configured within the backend program settings before initiating Google Pay™transactions. The domain should be provided without "https://", as the API handles secure connections internally. Merchants must align with their Product Solution Specialist to ensure all relevant domains are configured within the backend program settings before initiating Google Pay™transactions. The domain should be provided without "https://", as the API handles secure connections internally.
Please refer to Google Pay™ Console - Registration of the shop domain for more information.
Initiate Authorization Response
Status Code:
200 (Created)
Header:
Content-Type: application/json
Accept-Language: en-US
{
"programAccno": "7228894817",
"accno": "7228905548",
"uniqueReference": "KR3aoEVFDMGggM6Jt77J8",
"loadAccountReference": "5coZ0ldKLpOIUO3r6mCKA",
"authorizationToken": "eyJjb25uZW***AwMjZ2PTQifQ==",
"paymentOptionCode": "GGLPAY",
"presentationAmount": 100,
"presentationCurrCode": "EUR",
"presentationUsage": "V Purchase:2xPremiumWidgets. Merchant:WidgetsGmbH. CUSTREF:52650FD95.",
"statusCode": "RECEIVED",
"statusReason": "Successful transaction request.",
"paymentProviderResponse": {
"result": {
"kcAuthorizationToken": "eyJjb25uZW***AwMjZ2PTQifQ==",
"providerRequestDetails": {
"processedData": {
"authorizationToken": "936e***e86ccf",
"transactionData": {
"reference": "KR3aoEVFDMGggM6Jt77J8",
"providerReference": "",
"amount": 100,
"currency": "EUR",
"country": "",
"status": "RECEIVED",
"statusReason": "Successful transaction request."
}
},
"providerResponse": [],
"responseCode": "0000",
"responseDescription": "Operation succeeded.",
"localTime": "2025-01-28T12:58:50+01:00"
}
},
"responseCode": "0000",
"responseDescription": "Operation succeeded.",
"localDateTime": "2025-01-28T12:58:50+01:00",
"additionalData": [
{
"name": "externalCalls",
"value": [
{
"url": "{baseURL}/api/v1/payment/initAuthorize",
"httpMethod": "POST",
"httpStatusCode": 200
}
]
}
]
},
"partnerReference": "eb9640b0-f731-4abf-8752-37e8280c9ee9",
"localDate": "2025-01-28",
"localTime": "125849",
"sysDate": "2025-01-28",
"sysTime": "115850",
"responseCode": "0000",
"responseDescription": "Successful execution.",
"additionalInformation": {
"requestId": "46226f393fc247ee9b6439507ce382da"
}
}
The response includes the desired authorization token under the return parameter "authorizationToken" which is used to collect payment option details via the SDK and alongside the "uniqueReference" is required to complete the authorization of the initiated transaction. The transaction reference under the return parameter "uniqueReference" is furthermore required for the following API calls. Beyond this use, it should be persisted if possible, as it enables the identification of the transaction should the need arise at a later stage.
Please use the "authorizationToken" from the root level of the response, as other instances within nested structures are for internal purposes and should not be used for further API calls.
2. Collect Payment Option Details via KC UPCF Web SDK
The KC UPCF Web SDK renders a customizable Google Pay™ button, which can be customized in the previous step, and transmits all necessary payment details to start the Google Pay™ Checkout flow. We offer SDKs for the integration into websites as well as mobile applications. Google Pay™ authentication requires Google Wallet™ app on Android.
The example below shows only the relevant part of the KC UPCF Web SDK implementation for Guest Payments with Google Pay™. A complete and detailed description of the KC UPCF Web SDK can be found here.
Example code to handle KC UPCF Web SDK for Google Pay™ button:
window.kc.webSdk.PaymentForm(container, {
authorizationToken,
callbackUrl: `${window.location.origin}${completedOrder}`,
paymentOptionCodes: ['GGLPAY'],
locale: "en-US",
paymentProviderMode: "test",
submitButtonTitle: "buy",
onError: function (message) {
// handle errors
},
onComplete: function () {
// handle google authorization
}
})
The authorization token returned by the API method 1.38 Init Authorize which initiated the transaction is used to associate the payment option details collected via the KC UPCF Web SDK with the transaction.
3. Google Pay™ Checkout and Payment Confirmation
Following this, the user sees the confirmation screen, the chosen payment option and the amount to be authorized. Clicking the Pay button displays the payment details (see parameter presentationUsage
in the Initiate Authorization Request).
The maximum character length of the presentation usage (see variable presentationUsage
in the example above) varies between payment options. It may be that with certain payment options the specified presentation usage may be less than 127 and consequently be truncated. It would thus be strongly recommended that the most pertinent information be placed at the beginning of the presentation usage. To be compatible with most payment options we suggest that the presentation usage already be truncated at the 22nd character.
After confirming the payment with the Google Pay™ button, the KC UPCF Web SDK triggers the onComplete
function. This function is called upon successful payment processing, and merchants are responsible for defining what happens next. This can include displaying a confirmation message or redirecting the shopper to a success page. Please note that the KC UPCF Web SDK does not handle redirection automatically; any navigation must be implemented within the "onComplete" function.
Merchants should implement appropriate handling in the onComplete
and onError
callbacks to manage the user experience, such as retrying the process for errors or confirming the success of the transaction.
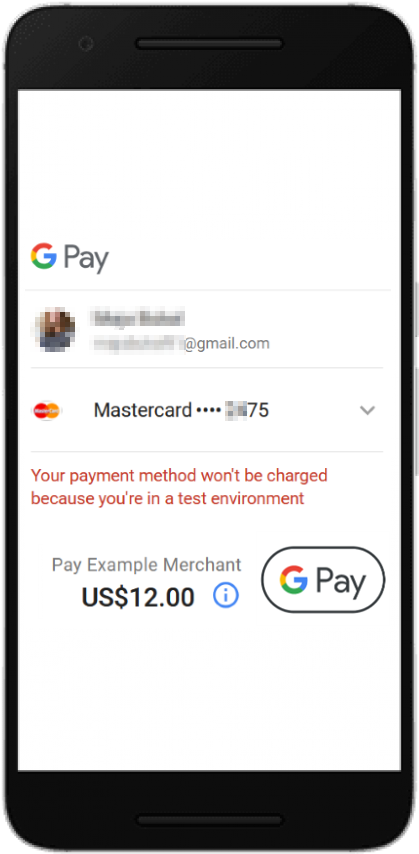
4. Complete Authorization
Since the KC UPCF Web SDK sends the payment details directly to Google Pay™, where the payment is subsequently processed, you have no direct visibility into the payment’s real-time authorization status. "onComplete" within the SDK indicates that the frontend component has successfully finished; however, this does not mark the final success of the payment.
Please note that KC UPCF Web SDK does not handle any redirection; it must be implemented in your custom logic.
After the onComplete
callback confirms successful payment processing, you should call the API method 1.39 Complete Authorize from your server-side application to finalize the transaction. A the payment is only considered fully successful once it has been successfully authorized, meaning the 1.39 Complete Authorize API response returns "responseCode": "0000"
or the status is Authorized
or Captured
. Please also see transaction status handling for non-successful response codes.
Merchants are responsible for defining the actions within the "onComplete" function, such as showing a confirmation page or initiating additional server-side processes.
Complete Authorization Request
Path:
POST {baseURL}/payment/{uniqueReference}/completeAuthorize
POST {baseURL}/payment/KR3aoEVFDMGggM6Jt77J8/completeAuthorize
Header:
Content-Type: application/json
Accept-Language: en-US
X-Auth-Token: uZWN0b3JCYXN***ODZjY2YifQ==
User-Agent: ***
{
"partnerReference": "eb9640b0-f731-4abf-8752-37e8280c9ee9",
"authorizationToken": "uZWN0b3JCYXN***ODZjY2YifQ==",
"localDate": "2025-01-28",
"localTime": "130137"
}
The transaction authorization is identified by the "uniqueReference" and "authorizationToken" returned initially by the API method 1.38 Init Authorize.
Complete Authorization Response
{
"initiatorAccno": "7228894817",
"accno": "7228905548",
"uniqueReference": "KR3aoEVFDMGggM6Jt77J8",
"processedAmount": 100,
"processedCurrCode": "EUR",
"statusCode": "AUTHORIZED",
"statusReason": "Authorization request has been approved by Acquirer/Payment provider and transaction can be requested for Capture.",
"paymentProviderResponse": {
"result": {
"providerRequestDetails": {
"processedData": {
"paymentOptionBrand": "GGLPAY",
"carrierNumber": "411111****1111",
"bic": "",
"cardExpiryMonth": "12",
"cardExpiryYear": "2027",
"eci": "",
"transactionData": {
"reference": "KR3aoEVFDMGggM6Jt77J8",
"providerReference": "8ac7a4a094a9c8450194acc9da6118ce",
"amount": 100,
"currency": "EUR",
"country": "",
"status": "AUTHORIZED",
"statusReason": "Authorization request has been approved by Acquirer/Payment provider and transaction can be requested for Capture."
},
"customerName": {
"fullName": "Demo Admin",
"firstName": "Demo",
"lastName": "Admin"
}
},
"providerResponse": [
{
"method": "POST",
"url": "{baseURL}/v1/payments",
"request": "amount=100¤cy=EUR&card.holder=Demo+Admin&card.number=41111111****1111&card.expiryMonth=12&card.expiryYear=2027&googlePay.source=web&paymentBrand=GOOGLEPAY&entityId=8ac7a4ca8b1e15be018b1e2918d80045&merchantTransactionId=KR3aoEVFDMGggM6Jt77J8&paymentType=PA&customer.browser.language=en-US&customer.browser.screenHeight=1080&customer.browser.screenWidth=1920&customer.browser.timezone=-60&customer.browser.userAgent=Mozilla%2F5.0+%28Windows+NT+10.0%3B+Win64%3B+x64%29+AppleWebKit%2F537.36+%28KHTML%2C+like+Gecko%29+Chrome%2F132.0.0.0+Safari%2F537.36+Edg%2F132.0.0.0&customer.browser.javaEnabled=false&customer.browser.javascriptEnabled=true&customer.browser.screenColorDepth=24&customer.browser.challengeWindow=05&shopperResultUrl=googlepay.jpmmps.com%2Fui%2Fv1%2Fredirect%3FauthorizationToken%3D936e1ceef3c***c6d0.8a2bac26***e999e86ccf",
"response": "{\"id\":\"8ac7a4a094a9c8450194acc9da6118ce\",\"paymentType\":\"PA\",\"paymentBrand\":\"VISA\",\"amount\":\"100\",\"currency\":\"EUR\",\"descriptor\":\"KR3aoEVFDMGggM6Jt77J8\",\"merchantTransactionId\":\"KR3aoEVFDMGggM6Jt77J8\",\"result\":{\"code\":\"000.100.110\",\"description\":\"Request successfully processed in 'Merchant in Integrator Test Mode'\"},\"card\":{\"bin\":\"411111\",\"last4Digits\":\"1111\",\"holder\":\"Demo Admin\",\"expiryMonth\":\"12\",\"expiryYear\":\"2027\"},\"customer\":{\"browser\":{\"language\":\"en-US\",\"screenHeight\":\"1080\",\"screenWidth\":\"1920\",\"timezone\":\"-60\",\"userAgent\":\"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/132.0.0.0 Safari/537.36 Edg/132.0.0.0\",\"javaEnabled\":\"false\",\"screenColorDepth\":\"24\",\"challengeWindow\":\"05\",\"javascriptEnabled\":\"true\"}},\"risk\":{\"score\":\"100\"},\"buildNumber\":\"c90a4b5aa476a11b858eb7abcd8ca88b9598d315@2025-01-27 00:42:27 +0000\",\"timestamp\":\"2025-01-28 12:01:37+0000\",\"ndc\":\"8ac7a4ca8b1e15be018b1e2918d80045_6cc96f2d8f624d62ba296a37360f2ed1\",\"source\":\"OPP\",\"paymentMethod\":\"CC\",\"shortId\":\"0299.7407.9181\"}",
"statusCode": "200"
}
],
"responseCode": "0000",
"responseDescription": "Operation succeeded.",
"localTime": "2025-01-28T13:01:38+01:00"
}
},
"responseCode": "0000",
"responseDescription": "Operation succeeded.",
"localDateTime": "2025-01-28T13:01:38+01:00",
"additionalData": [
{
"name": "externalCalls",
"value": [
{
"url": "{baseURL}/api/v1/payment/completeAuthorize",
"httpMethod": "POST",
"httpStatusCode": 200
}
]
}
]
},
"partnerReference": "eb9640b0-f731-4abf-8752-37e8280c9ee9",
"localDate": "2025-01-28",
"localTime": "130137",
"sysDate": "2025-01-28",
"sysTime": "120138",
"responseCode": "0000",
"responseDescription": "Successful execution.",
"additionalInformation": {
"requestId": "6600d2fc4fe74281855a63f09a920043"
}
}
Note, that the 1.39 Complete Authorize response includes the internal representation of the Account Number indicated by the parameter Account Number Type. Additionally, the response includes the transaction status under the status code parameter, which, at this stage, should be set to AUTHORIZED
, indicating that the payment has been successfully authorized.
Please also see transaction status handling for non-successful response codes.